About this course
The "Data Structures and Algorithms (DSA)" course is a comprehensive, 30-hour program designed to equip students with a solid foundation in the essential concepts of data structures and algorithms. This course is tailored for individuals looking to enhance their problem-solving skills and understanding of efficient algorithmic design, making it ideal for those aspiring to excel in technical interviews, competitive programming, and software development.
What You Will Learn:
1. Basic Data Structures:
Arrays: Learn the fundamentals of arrays, including memory allocation and operations like insertion, deletion, and traversal. Explore multi-dimensional arrays and their applications in real-world scenarios.
Linked Lists: Dive deep into linked lists, understanding various types such as singly, doubly, and circular linked lists. Master advanced operations like reversing a linked list and detecting cycles.
Stacks and Queues: Understand stack and queue data structures, their operations, and applications in solving problems like expression evaluation and job scheduling. Get hands-on experience with advanced concepts like circular and priority queues.
Trees and Graphs: Explore the world of trees, including binary trees, binary search trees (BST), and AVL trees. Learn about graph theory, including BFS and DFS, and their applications in networking, social networks, and more.
2. Algorithms:
Sorting and Searching Algorithms: Master essential sorting algorithms like Bubble Sort, Merge Sort, and Quick Sort, and search algorithms like Linear Search and Binary Search. Learn to analyze and optimize their performance using time and space complexity.
Recursion and Dynamic Programming: Understand the power of recursion in solving complex problems and transition to dynamic programming to optimize solutions using memoization and tabulation techniques.
Greedy Algorithms and Backtracking: Discover the efficiency of greedy algorithms in solving problems like the Knapsack and Job Scheduling. Explore backtracking techniques to solve puzzles and generate permutations and combinations.
3. Problem-Solving Techniques:
Coding Practice: Enhance your problem-solving skills by practicing on platforms like LeetCode and HackerRank. Tackle real-world problems involving arrays, linked lists, stacks, and queues, and receive guidance on improving your approach.
Complexity Analysis: Develop a deep understanding of time and space complexity, learning to evaluate and optimize algorithms for better performance. Analyze case studies to see how these concepts apply to real-world problems.
Who Should Enroll:
This course is perfect for students, software developers, and anyone interested in strengthening their understanding of data structures and algorithms. Whether you’re preparing for technical interviews, looking to compete in coding challenges, or simply aiming to improve your programming skills, this course provides the necessary tools and knowledge to succeed.
Course Format:
The course combines interactive lectures, hands-on coding exercises, and problem-solving sessions. Each topic is presented with clear explanations, followed by practical examples and exercises to reinforce learning. By the end of the course, students will have a robust understanding of DSA and the confidence to apply these concepts in real-world scenarios.
Course Outcomes:
1. Gain proficiency in essential data structures like arrays, linked lists, stacks, queues, trees, and graphs.
2. Master key algorithms, including sorting, searching, recursion, dynamic programming, and backtracking.
3. Develop the ability to analyze and optimize algorithms based on time and space complexity.
4. Build problem-solving skills by practicing on leading coding platforms.
5. Be well-prepared for technical interviews and competitive programming challenges.
FAQ
Comments (0)
Overview of arrays, memory allocation, and basic operations (insertion, deletion, traversal).
: Types (Singly, Doubly, Circular), operations (insertion, deletion, traversal).
Stack operations (push, pop, peek), applications (expression evaluation, backtracking).
Binary Trees, Binary Search Trees (BST), tree traversal methods (in-order, pre-order, post-order).
Bubble Sort, Selection Sort, Insertion Sort, practical implementation.
Basic recursive algorithms, understanding base cases, and recursive cases.
Concepts, applications, solving problems like Fractional Knapsack, and Job Scheduling.
Overview of LeetCode, HackerRank, setting up profiles, and selecting problems.
Time complexity analysis of basic algorithms.

Reviews (1)
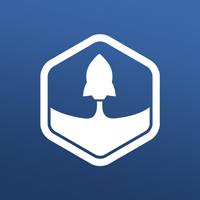